6.2. namedtuplex.abc¶
The namedtuplex.abc module provides the enhanced replacement of containers.namedtuple. The namedtuplex.abc.namedtuple is drop-in compatible with the containers.namedtuple interface. The contained public classes interfaces are:
Alias for NamedTupleXABC.
Abtstract base class with support of inheritance and mixin.
The contained secondary public utility classes. These serve as internal template and metaclass, which could be alternated by call interface parameters.
Alias for NamedTupleXABCMeta.
Meta class for the parameterized creation.
The common return value for the interfaces is a namedtuple class of the type defined by the input parameter typename. For a detailed overview refer to “The namedtuplex API - Parameters”.
6.2.1. Inheritance¶
The class NamedTupleXABC supports the inheritance by derived classes. The class itself is an abstract class based on the metaclass NamedTupleXABCMeta.
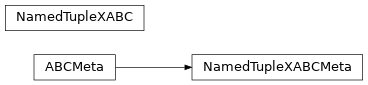
The class supports the inheritance from abstract classes as well as from non-abstract classes.
6.2.2. Mixin¶
The class NamedTupleXABC supports the mixin by derived classes.
6.2.3. Module¶
The package ‘namedtuplex’ provides an extended call interface and classes for namedtuples.
6.2.4. Functions¶
6.2.4.1. with_metaclass¶
The function with_metaclass is copied from future.utils [future.utils].
-
namedtuplex.abc.
with_metaclass
(meta, *bases)[source]¶ - Function from future/utils.py License: BSD.
Function from jinja2/_compat.py. License: BSD.
Do need a wrapper only for the compilation-breaking metaclass syntax of Python2/Python3. Require this on all platforms - including Jython.
Original doc-string:
Function from jinja2/_compat.py. License: BSD.
Use it like this:
class BaseForm(object): pass class FormType(type): pass class Form(with_metaclass(FormType, BaseForm)): pass
This requires a bit of explanation: the basic idea is to make a dummy metaclass for one level of class instantiation that replaces itself with the actual metaclass. Because of internal type checks we also need to make sure that we downgrade the custom metaclass for one level to something closer to type (that’s why __call__ and __init__ comes back from type etc.).
This has the advantage over six.with_metaclass of not introducing dummy classes into the final MRO.
6.2.5. ABC¶
-
namedtuplex.abc.
ABC
¶ Reference to the abstract base class.
alias of
namedtuplex.abc.NamedTupleXABC
Same as namedtuple.abc.NamedTupleXABC.
6.2.6. NamedTupleXABC¶
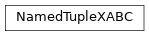
-
class
namedtuplex.abc.
NamedTupleXABC
[source]¶ The abstract class for extended tuple classes. The metaclass syntax is based on future.utils [future.utils] with support for both Python syntax variants of Python2.7 and Python3. For the metaclass refer to NamedTupleXABCMeta.
-
_fields
¶ The abstract property, see abc.abstractproperty [abc], and fields and _fields.
-
_fielddefaults
= None¶ Optional default values for _fields in function-paramater style see fielddefaults and _fielddefaults.
-
6.2.6.1. merge¶
-
NamedTupleXABC.
merge
(*others, **kargs)[source]¶ Creates a new instance by concatenating the others to the copy of current instance created with standard members. Additional attributes, properties, and methods have to be processed by derived classes.
- Parameters
others –
Instances of tuples to be merged into this instance. The merge is processed by right-hand concatenation of others.
- The supported types are:
NamedTupleXABC OrderedDict # same as from '_asdict()' <namedtuple> # the result of 'namedtuple()' tuple # when 'rename' is 'True'
kargs –
- deep:
If True merges a deep copy of all, else a swallow copy only.
default := False
- module:
Optional parameter to be passed to the tuplefactory.
default := None
- rename:
Optional parameter to be passed to the tuplefactory.
default := None
- tuplefactory:
The tuplefactory callable to be used for the new named tuple.
default := namedtupledefs.namedtuple
- verbose:
Optional parameter to be passed to the tuplefactory.
default := None
- Returns
A new instance of merged objects.
- Raises
ValueError –
pass-through –
6.2.6.2. inherited tuple members¶
Inherited members from the parent namedtuple, by default namedtupledefs.namedtuple.
_make
_asdict
_replace
__repr__
__getnewargs__
__getstate__
merge
6.2.7. NamedTupleXABCMeta¶

-
class
namedtuplex.abc.
NamedTupleXABCMeta
[source]¶ The metaclass for named tuples as an abstract base with support for inheritance and mixin. For the abstract class refer to NamedTupleXABC.
6.2.7.1. __new__¶
-
static
NamedTupleXABCMeta.
__new__
(cls, name, bases, namespace, **kargs)[source]¶ Adds the symbolic field names for the indices and selected attributes as member variables.
- Parameters
cls – The created class.
name – The name of the created class.
bases – List of base classes.
namespace – The namespace, containing the _fields class variable.
kargs –
- fields:
The fields parameter provides a list of alternate names for the contained tuple members. This in advance defines the required mandatory number of items for the tuple.
fields := ( '"' <index-names> '"' | '[' <index-list> ']' | '(' <index-list> ')' ) index-names := <index-name>[[ ,] <index-names>] index-list := <index-name>[, <index-list>] index-name := "valid name for the field index of the tuple"
- fielddefaults:
Defines default values for the fields of the tuple. This allows for the variable length initialization of the created namedtuple class.
fielddefaults := '(' <default-list> ')' default-list := '(' <key> ',' <value> ')' key := ( <field-index> | <field-name> ) field-index := "valid integer index of a present field" field-name := "valid name from the list _fields" value := "default value"
Default values are supported in accordance to the standard Python behaviour of call parameters [PYFUNC].
- module
Sets __module__ of the created class definition.
Available beginning with Python-3.6. See [namedtuple].
- tuplefactory:
The class factory to be used for the named tuple.
default := collections.namedtuple
- rename:
If True replaces silently invalid field names by ‘_<item-index>’.
default := False
Available Python-2.7 and in Python-3.x see manuals “…beginning with Python3.1”. See [namedtuple].
- useslots:
Creates a member __slots__.
default := True
See [namedtuple].
- verbose:
Prints created class definition.
default := False
See [namedtuple].
- Returns
An abstract class derived from namedtuple with alternative symbolic names for the contained items. In addition predefined attributes are included.
- Raises
pass-through –
Examples:
Python3: class _NamedTupleABC(metaclass=NamedTupleXABCMeta): _fields = abstractproperty() attribute01 = default01 attribute02 = default02 # common part for Python3 and Python2 def __new__(cls, *args, **kargs): try: attribute01 = kargs.pop('attribute01') except KeyError: attribute01 = default01 try: attribute02 = kargs.pop('attribute02') except KeyError: attribute02 = default02 return super(_NamedTupleABC, cls).__new__(cls, *args, **kargs) Python2: class _NamedTupleABC(object): __metaclass__ = NamedTupleXABCMeta _fields = abstractproperty() attribute01 = default01 attribute02 = default02 # common part - see Python3 ...